1. 기본 스크립트
using System.Collections; using System.Collections.Generic; // Data Type using UnityEngine; // 유니티가 동작하는 데 필요한 기능 제공 public class Test : MonoBehaviour // c#으로 작성한 프로그램은 클래스 단위로 관리. // 클래스명 == 스크립트명 { // Start is called before the first frame update void Start() { //캐릭터를 표시하는 처리 } // Update is called once per frame void Update() { //현재 캐릭터를 조금씩 오른쪽으로 옮기는 처리 //캐릭터 표시 뿐만 아니라 충돌 판정이나 키 조작 등의 처리를 프레임마다 해야 함. } }
✅ FPS(Frame Per Second) : 1초에 표시되는 프레임 매수를 뜻합니다.
🎬영화는 1초에 24프레임(24FPS),
🎮게임은 1초에 60프레임(60FPS) 속도로 그림을 전환해 애니메이션을 만들죠.
Unity에서 실제로 앞의 프레임부터 몇 초 지났는 지는 Time.deltaTime 구조로 알 수 있습니다.
💡스크립트를 실행시키면 Start 메서드는 한 번만 실행되지만 Update 메서드는 프레임마다 반복해서 실행됩니다. 예를 들어 캐릭터가 오른쪽으로 걸어가는 애니메이션을 만든다면 처음에는 Start 메서드로 캐릭터를 표시하고, 그 다음에는 Update 메서드로 프레임마다 캐릭터를 오른쪽으로 옮기는 처리를 할 수 있죠.
✅ 스크립트의 큰 흐름은 다음과 같습니다.
1. 스크립트 실행
2. Start (한 번만 실행)
3. Update (한 프레임마다 실행)(프레임마다 반복)
2. C# 문법 연습_1
using System.Collections; using System.Collections.Generic; using UnityEngine; public class Test : MonoBehaviour { // Start is called before the first frame update void Start() { Debug.Log("Hello Yeonhee"); int age; age = 25; Debug.Log(age); float height1 = 160.5f; float height2; height2 = height1; Debug.Log(height2); string name; name = "Sera"; Debug.Log(name); int answer; answer = 1 + 2; Debug.Log(answer); answer = 3 - 4; Debug.Log(answer); answer = 5 * 6; Debug.Log(answer); answer = 8 / 4; Debug.Log(answer); } }
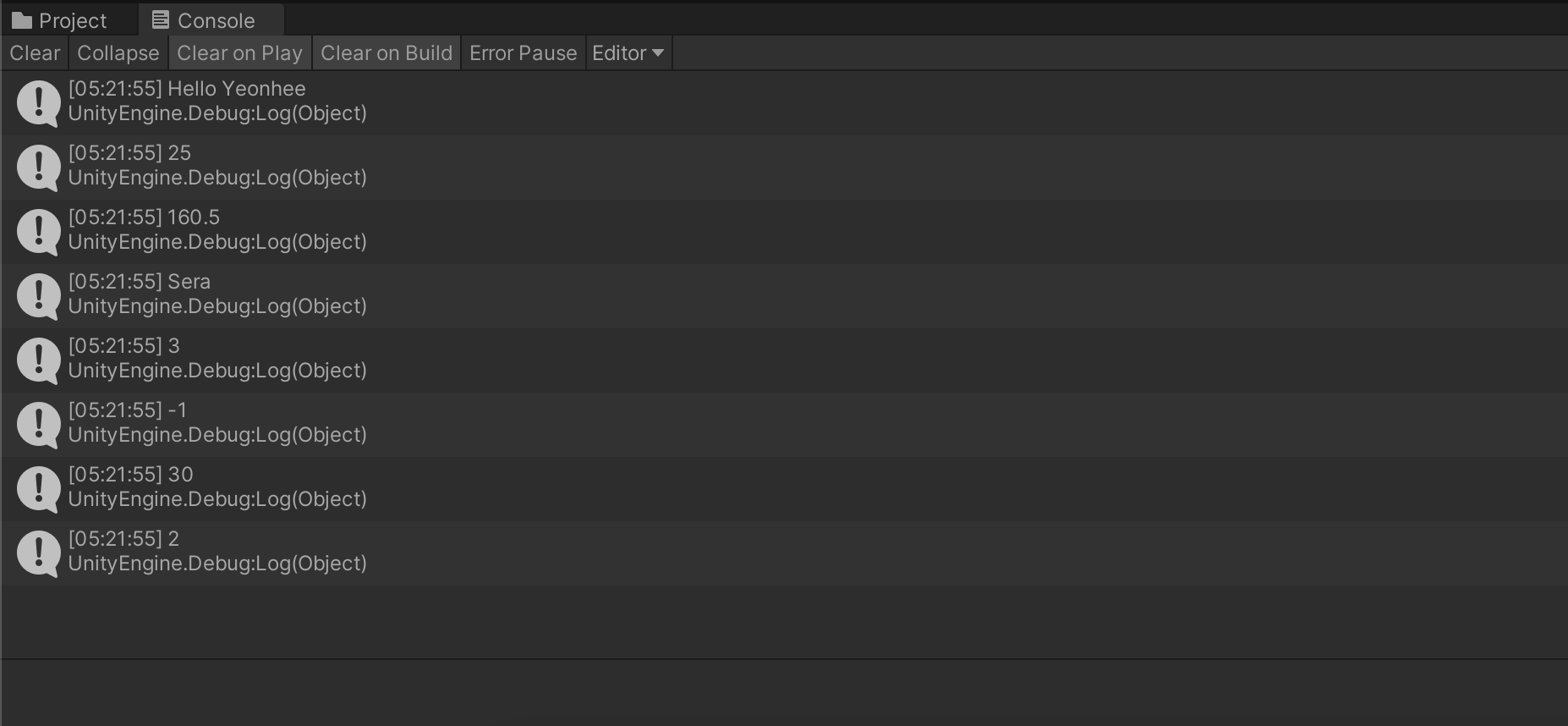
3. C# 문법 연습_2
using System.Collections; using System.Collections.Generic; using UnityEngine; public class Test : MonoBehaviour { // Start is called before the first frame update void Start() { int n1 = 8; int n2 = 9; int answer1; answer1 = n1 + n2; Debug.Log(answer1); int answer2 = 10; answer2 += 5; Debug.Log(answer2); int answer3 = 10; answer3++; Debug.Log(answer3); string str1 = "merry "; string str2 = "christ mas"; str1 += str2; Debug.Log(str1); string str = "happy "; int num = 123; string message = str + num; Debug.Log(message); } }
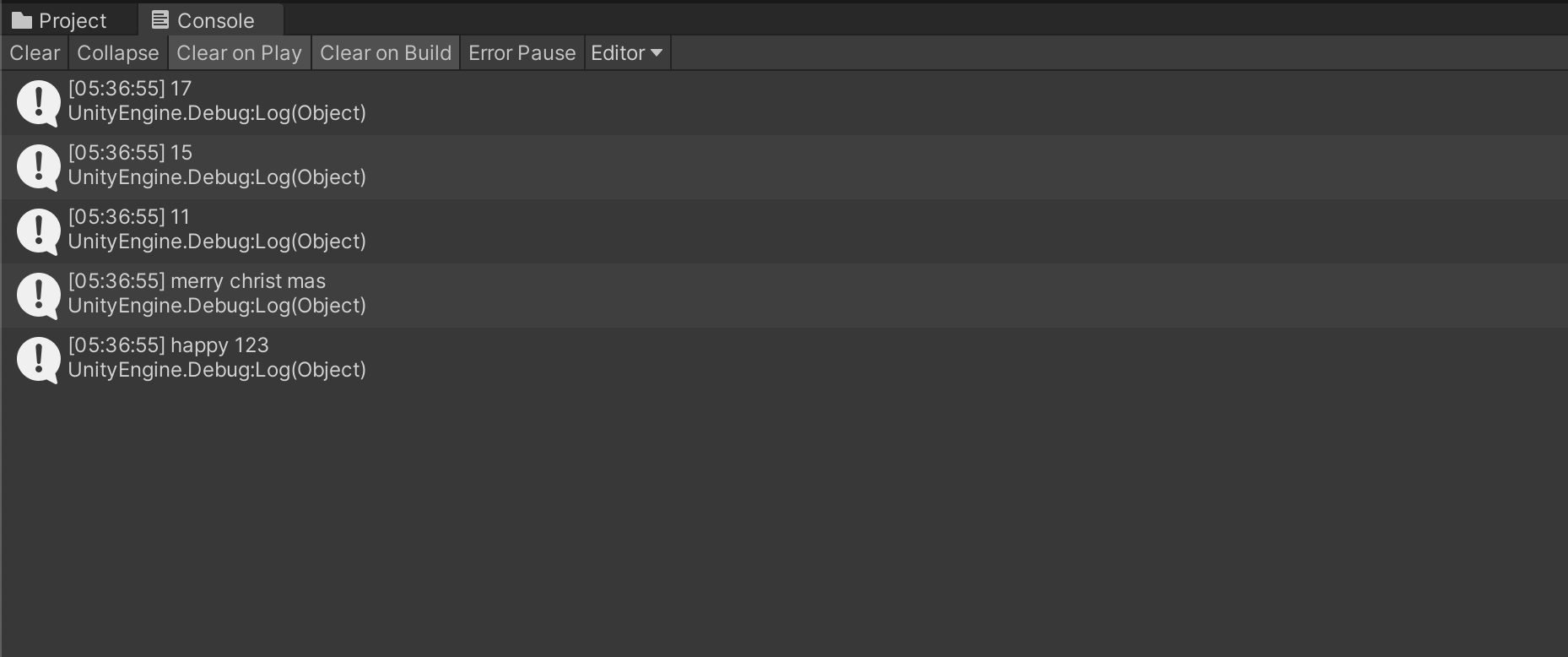
'game as a service' 카테고리의 다른 글
Google I/O 2019 Codelab 중 Unity와 Firebase를 활용하는 실습 (0) | 2021.01.01 |
---|---|
유니티 연습 5 : Vector 클래스 (0) | 2020.12.27 |
유니티 연습 4 : 클래스 (0) | 2020.12.27 |
유니티 연습 3 : 메서드(함수) (0) | 2020.12.27 |
유니티 연습 2 : 배열 (0) | 2020.12.27 |